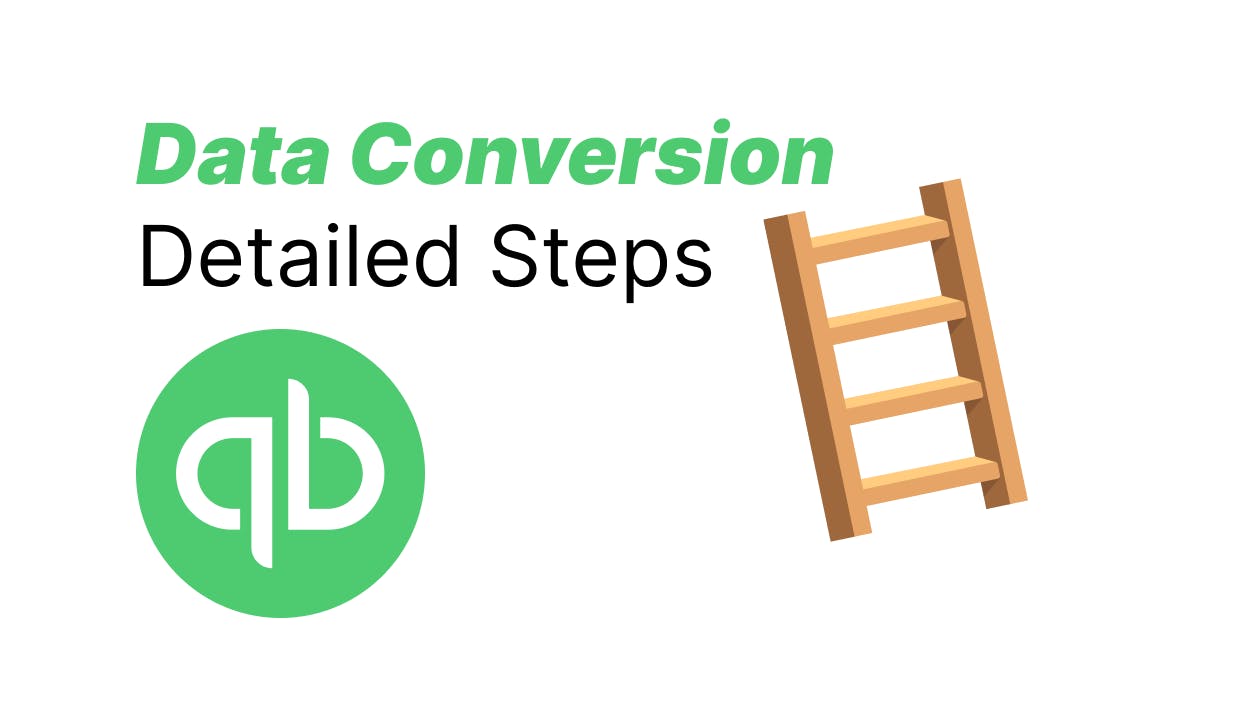
Learn how to authenticate QuickBooks Online users in your Intuit App using OAuth 2.0 with this simple tutorial. Works with Express, Nextjs and Sveltekit.
Integrating QuickBooks Online API in a SvelteKit app involves several steps, including setting up OAuth for authentication, obtaining an access token, and using it to interact with the QuickBooks API. Let’s break down the code and address potential issues and necessary steps to get it working.
You have this part set up correctly. Ensure your environment variables are properly set.
import type { LayoutServerLoad } from './$types';
import OAuthClient from 'intuit-oauth';
import { CLIENT_ID, CLIENT_SECRET, ENVIRONMENT, REDIRECT_URI } from '$env/static/private';
export const load = (async ({ locals }) => {
// Initialize OAuthClient
const oauthClient = new OAuthClient({
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
environment: ENVIRONMENT,
redirectUri: REDIRECT_URI
});
// Generate the auth URI
const authUri = oauthClient.authorizeUri({
scope: [OAuthClient.scopes.Accounting, OAuthClient.scopes.OpenId],
state: 'Init'
});
return { authUri }; // Return the authUri for redirection
}) satisfies LayoutServerLoad;
Redirect users to authUri to start the OAuth flow:
<a href={$authUri}>Connect to QuickBooks</a>
You need to create a route to handle the QuickBooks redirect:
// src/routes/api/callback/+server.ts
import { json } from '@sveltejs/kit';
import OAuthClient from 'intuit-oauth';
import { CLIENT_ID, CLIENT_SECRET, ENVIRONMENT, REDIRECT_URI } from '$env/static/private';
/**
* Retrieves an authorization token from the OAuthClient and returns a JSON response with the name 'Aditya'.
*
* @param {Object} param - An object containing the URL parameter.
* @param {URL} param.url - The URL object containing the query parameters.
* @return {Promise<Object>} A Promise that resolves to a JSON object with the name 'Aditya'.
*/
export async function GET({ url }) {
const oauthClient = new OAuthClient({
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
environment: ENVIRONMENT,
redirectUri: REDIRECT_URI
});
// Get the authorization code from query parameters
const authCode = url.toString();
console.log('authCode', authCode);
const tokenExchange = await oauthClient.createToken(authCode);
console.log('exchange res', tokenExchange);
return json({ status: 200 });
}
With the access token, you can now make requests to QuickBooks API:
// src/routes/api/data/+server.ts
import { json } from '@sveltejs/kit';
import OAuthClient from 'intuit-oauth';
import { CLIENT_ID, CLIENT_SECRET, ENVIRONMENT, REDIRECT_URI } from '$env/static/private';
// Assuming you have stored the token securely
// const token = getStoredToken();
export async function GET({ locals }) {
const oauthClient = new OAuthClient({
clientId: CLIENT_ID,
clientSecret: CLIENT_SECRET,
environment: ENVIRONMENT,
redirectUri: REDIRECT_URI
});
// Set token in oauthClient
oauthClient.setToken(locals.token);
try {
// Example API request to get company info
const companyInfo = await oauthClient.makeApiCall({
url: `${oauthClient.environment === 'sandbox' ? 'https://sandbox-quickbooks.api.intuit.com' : 'https://quickbooks.api.intuit.com'}/v3/company/YOUR_COMPANY_ID/companyinfo`
});
return json(companyInfo.getJson());
} catch (error) {
console.error('API call error:', error);
return json({ error: 'API call failed' }, { status: 500 });
}
}
OAuth 2.0 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service. It works by delegating user authentication to the service that hosts the user account and authorizing third-party applications to access the user account.
To set up OAuth 2.0 with Intuit on a Node.js server, you need to install the `intuit-oauth` package, initialize the OAuth client with your credentials, generate the authorization URL, and handle the token exchange upon user redirection.
Yes, you can use OAuth 2.0 with both Next.js and SvelteKit. For Next.js, you can set up API routes to handle the OAuth flow. For SvelteKit, you can use server-side load functions and API routes to manage authentication and token exchange.
For OAuth 2.0 integration with Intuit, you need the following environment variables: `CLIENT_ID`, `CLIENT_SECRET`, `ENVIRONMENT`, and `REDIRECT_URI`. These should be securely stored and not exposed in client-side code.
The key steps include: initializing the OAuth client, generating the authorization URL, redirecting users to QuickBooks for authorization, handling the callback to exchange the authorization code for an access token, and using the access token to make authenticated API requests.